This lambda will trigger the second lambda.
Step 1 - Configure IAM
First create an IAM role that will allow you to not only trigger a lambda but also allow one lambda to trigger another lambda.
My role will be named role-lambda-basic-execution
.
Step 2 - Create Lambda Function #1
This lambda function is actually pretty handy. If you provide it with an IP address, it will respond back with the geo location (lat, lng). I will name it TrackIp
.
The code is written in ECMA Script 6 for Node v8.10.
exports.handler = async (event) => {
// TODO implement
return 'Hello from Lambda!'
};
Step 3 - Create Lambda Function #2 (Caller)
Similar to the functin above, I will create a new method named caller
.
var aws = require('aws-sdk');
var lambda = new aws.Lambda({
region: 'us-west-2' //change to your region
});
exports.handler = async (event, context, callback) => {
lambda.invoke({
FunctionName: 'arn:aws:lambda:us-west-2:xxxxxx:function:TrackIP',
Payload: JSON.stringify(event, null, 2)
}, function(error, data) {
if (error) {
context.done('error', error);
}
if(data.Payload){
context.succeed(data.Payload)
}
});
};
Resources
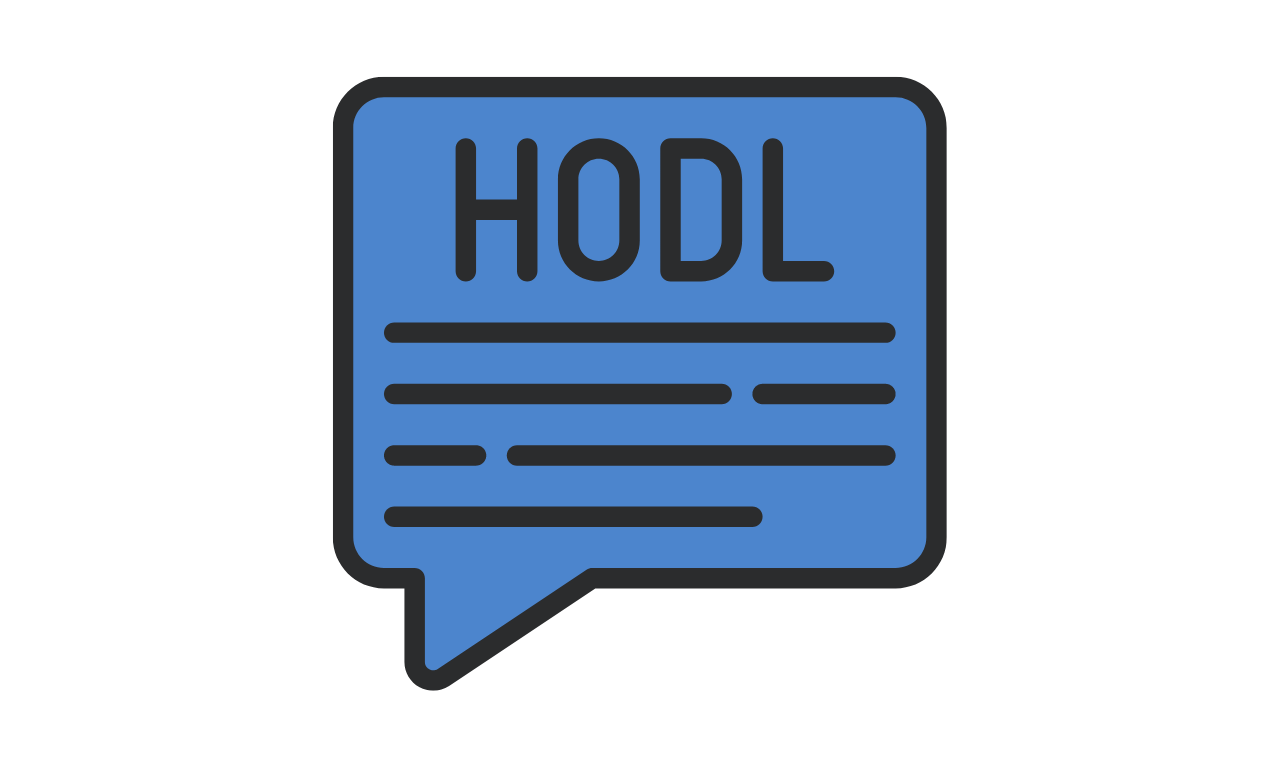
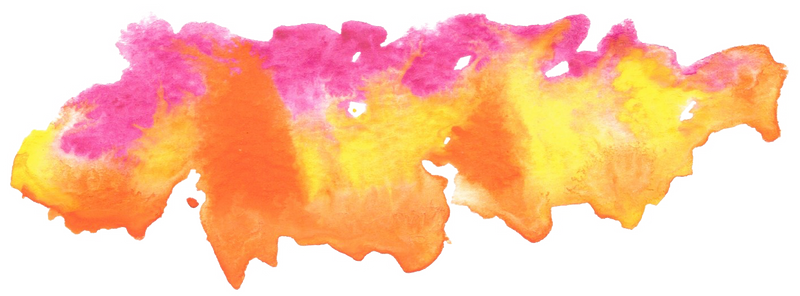
Deploying Rails 5.x on AWS ElasticBeanstalk using AWS CodeCommit
How to deploy your Rails app on ElasticBeanstalk (including S3 buckets, security groups, load balancers, auto-scalling groups and more) using CodeCommit.
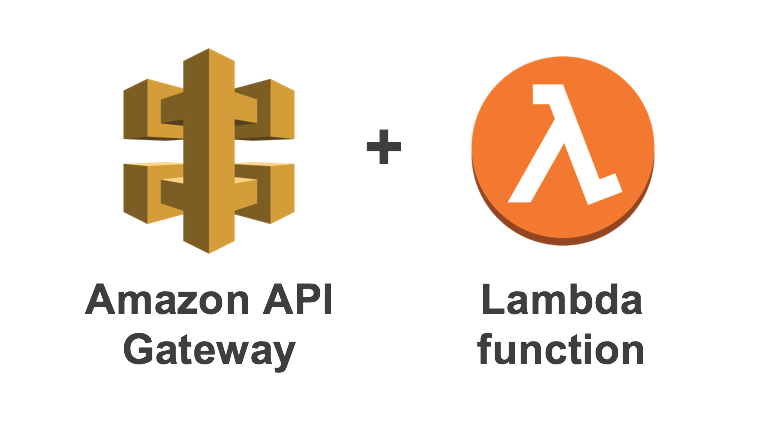
Subscribe to new posts
Processing your application
Please check your inbox and click the link to confirm your subscription
There was an error sending the email