Here's how I convert MOV files into animated GIF's for quick preview and e-mail friendly sharing. My source code is available as a Github repo on Github.
Note: This tutorial was written for developers using Mac OS X.
Getting Started
Install Homebrew
Homebrew is a package manager for Mac OS x. You will need this to later download a few open source libraries.
Install FFmpeg
Using homebew, you will need to install ffmpeg
. This is the tool that will compress your existing .mov file.
brew install ffmpeg
Install Quartz
Quartz is required to use the next package, Gifsicle.
brew install xquartz --cask
Install Gifsicle
brew install gifsicle
Run Ruby Script
I created a Convert class that will do all the work. I made it Object Oriented so that you can later decide to integrate it into a larger application.
APP_ROOT = File.dirname(__FILE__)
class Converter
# Default path to ffmpeg installed by homebrew
@@ffmpeg = File.join('/', 'usr', 'local', 'bin', 'ffmpeg')
# Default path to gifsicle installed by homebrew
@@gifsicle = File.join('/', 'usr', 'local', 'bin', 'gifsicle')
# Tell ffmpeg the max-width and max-height
@@max_size = "1280x1024"
# Tell ffmpeg to reduce the frame rate from to 30
@@frames = "30"
# Tell gifsicle to delay 30ms between each gif (in ms)
@@delay = 3
# Requests that gifsicle use the slowest/most file-size optimization
@@optimize = 3
def initialize(input, output)
shell_command = %{#{@@ffmpeg} -i #{input} -s #{@@max_size} -pix_fmt rgb24 -r #{@@frames} -f gif - | #{@@gifsicle} --optimize=#{@@optimize} --delay=#{@@delay} > #{output}}
# puts command
%x[ #{shell_command} ]
end
end
# Be careful not to use spa ces.
input = File.join(APP_ROOT, 'media', 'Circle.mov')
output = File.join(APP_ROOT, 'media', 'Circle.gif')
Converter.new(input,output)
Using this simple class as my base, I can easily integrate this into a Rake Task or maybe use FileList
to do more work with string manipulation. Hopefully this simple tutorial shows you that the possibilities are infinite using Ruby, Shell, and home-brew.
Final Thoughts
If you care about aesthetics, you can tweak the parameters until there's something you like. The nice part is that the Ruby class makes it very easy to read and modify.
Resources
- My Github Source Code
- If you want to learn more about how
%x
works in Ruby, read this tutorial
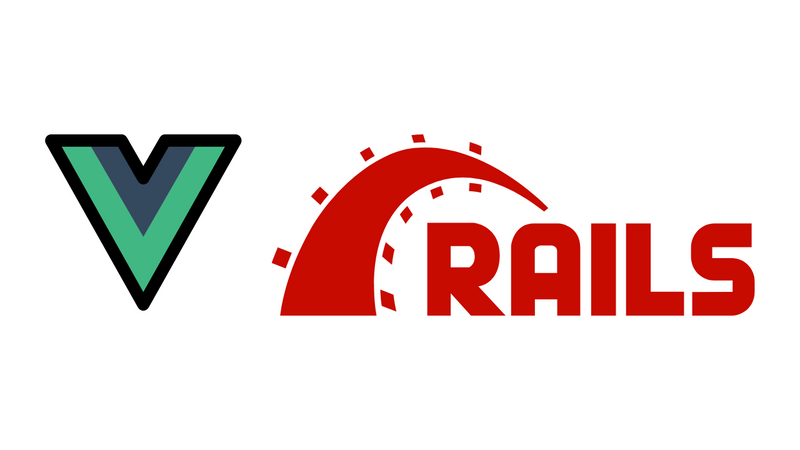
Deploying Rails 5.x on AWS ElasticBeanstalk using AWS CodeCommit
How to deploy your Rails app on ElasticBeanstalk (including S3 buckets, security groups, load balancers, auto-scalling groups and more) using CodeCommit.
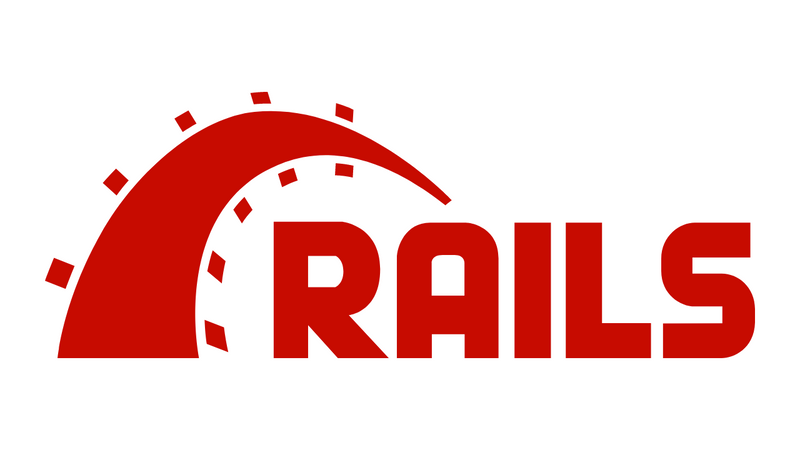